Let's create an MPAGD game - Part 19: The other blocks: DEADLY, CUSTOM and WATER
- Bruce
- Oct 17, 2021
- 7 min read
Updated: Oct 30, 2021
Let's finish off learning about the different types of blocks we can use to design our games screens by looking at the ones we haven't used yet:
DEADLYBLOCKs
CUSTOMBLOCKs
WATERBLOCKs
DEADLY BLOCKS
In general we use DEADLYBLOCKS when we want to inflict damage or kill the player if they touch them.
Let's create some deadly spikes... open up the Block Editor and press X to add a new block:
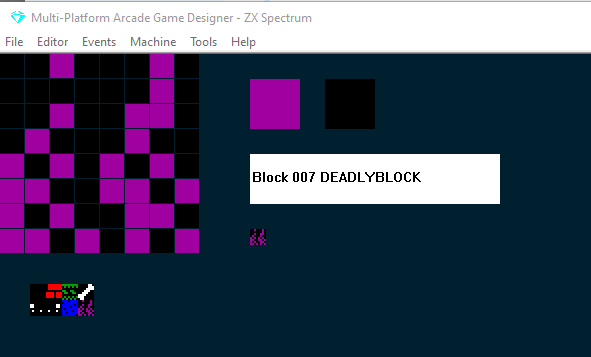
Design your DEADLYBLOCK and keep clicking the white block type selector to cycle through to DEADLYBLOCK.
Next, we'll add some deadly blocks to a screen, from the Editor menu select screens, then click your deadly block in the block bank and click to place some in your screen:
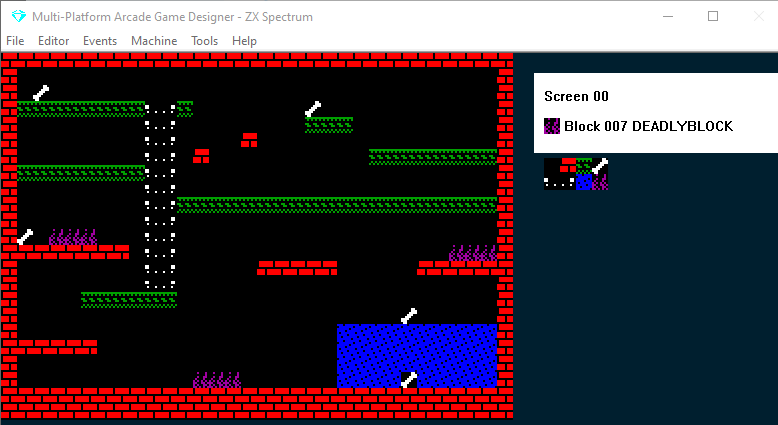
Next, take a look in your PLAYER EVENT code, you should find a statement that looks like this:
IF DEADLY ; am I touching something deadly?
KILL ; if so, kill me
ENDIF
DEADLY is a function, it tests to see if the player is touching a DEADLYBLOCK and if so, it will run whatever is contained within the IF...ENDIF statement - as standard, this will be KILL
BUT IT DOESNT HAVE TO! you could change this, in fact you can do whatever you like with it...it doesn't even have to be something bad or damaging to the player!
For now though, we'll leave it as it is and test our game.
So now Stinky Dog will die if he touches the deadly spikes.
CUSTOMBLOCKs
In many respects CUSTOMBLOCKs operate exactly the same as DEADLYBLOCKS, they too have a corresponding function which we can test for using IF CUSTOM.
All you need to do is write some code that will activate when the player touches a CUSTOMBLOCK.
Let's create some slippery ice, that if Stinky Dog stands on he will slide across, open up the BLOCK EDITOR, hit X for a new block and design some ice. Then click through the white blocktype selector box and stop at CUSTOMBLOCK:
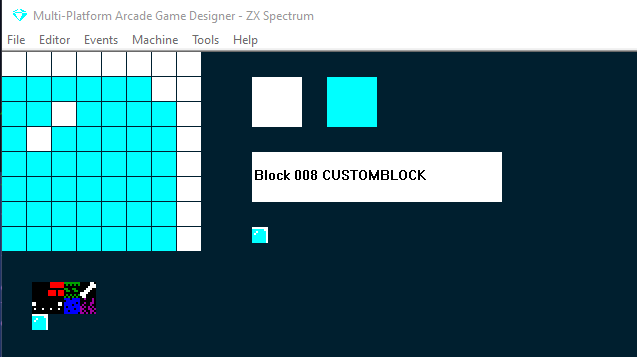
Let's now add a row of these custom ice blocks to our screen: (Editor > Screens )
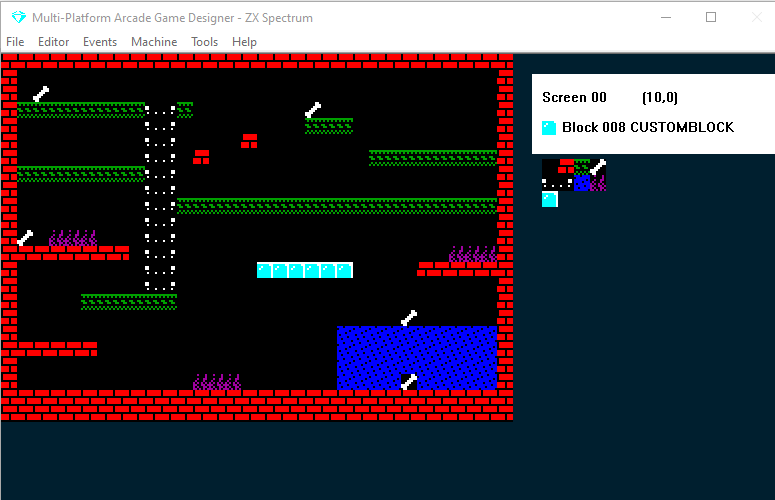
And before we write any code for what should happen, let's see what happens by default, test your game:
As you can see, as standard, CUSTOMBLOCKs are like EMPTYBLOCKS, the player just passes through them - which kind of makes sense, as we can use them for whatever purpose we like. But in our game, ideally we want them to be like WALLBLOCKS, the player cant pass through them but can stand on them...but then have a special property that forces the player to move in the direction they are facing.
First, let's see if we can achieve this by writing some code. We could try the STOPFALL command, this will stop the player from falling through them. Then, as we are storing the players current direction in the DIRECTION variable we could write some IF DIRECTION = statements to force movement...it might look something like this:
IF CUSTOM ; if player touches the ice
STOPFALL ; stop them falling
IF DIRECTION = LEFT ; if they were travelling left
SPRITELEFT ; go left
SPRITELEFT ; go left some more
ENDIF
IF DIRECTION = RIGHT ; if they were going right
SPRITERIGHT ; go right
SPRITERIGHT ; go right some more
ENDIF
ENDIF
Note that I've doubled up on the SPRITELEFT/RIGHTs - this should give the effect of faster movement when on the ice. Let's give it a test:
OK, it kind of works, the first time I jumped on the ice I slid across to the left, but when I hit the ice from beneath I slid to the right by my head. Then I jumped back on and used the LEFT and RIGHT keys and Stinky dog slid from side to side.
The IF CUSTOM function only knows I am touching a custom block, it doesn't know it is ice and that I cant slide underneath it.
The trick to solve this issue in our game is to switch the ice blocks to WALLBLOCKS and then create a new CUSTOM block that we will place above the ice WALLBLOCKS. Before we test it, we'll remove the STOPFALL command from the IF CUSTOM code that we just wrote. Let's give it a test:
Change the ice block to a WALLBLOCK:

Create a new custom block (I've added the word ICE on it so you can see it when we place it on the screen...we'll remove it later):

Add your new custom blocks above the ice
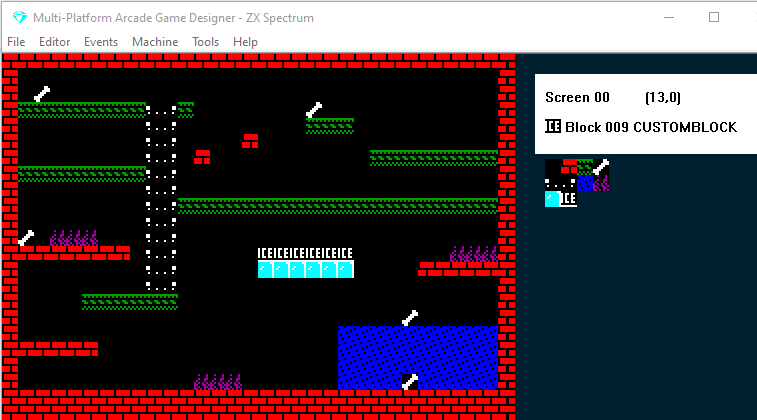
And give it a test:
OK, It's getting better, now stinky dog is sliding on the ice, he also can't slide underneath it. He can, however still move from left to right using the LEFT and RIGHT keys. This might be OK for your game, but I want to force the movement and not allow him to move or jump when on the ice.
And the solution to this is quite easy, all we need to do is wrap all of our IF KEY statements inside the ELSE part of:
IF CUSTOM
{force the sliding on the ice}
ELSE
{all the if key statements go here}
ENDIF
What this means is : as long as we are not touching a custom block you can use the keys to move. Let's give this a test (note that I have removed the word ICE from my custom block and left the single row of white pixels at the bottom of it:
To check your progress, here's what your PLAYER EVENT code should look like by now:
EVENT PLAYER
;===============STINKY DOG=================
IF CUSTOM
IF DIRECTION = LEFT ; if they were travelling left
SPRITELEFT ; go left
SPRITELEFT ; go left some more
ENDIF
IF DIRECTION = RIGHT ; if they were going right
SPRITERIGHT ; go right
SPRITERIGHT ; go right some more
ENDIF
ELSE
IF KEY LEFT ; Is the left key pressed?
LET DIRECTION = LEFT ; if so, remember I am going LEFT
LET IMAGE = 1 ; display the left facing image
IF X <= LEFTEDGE ; are we at the left edge of the screen?
SCREENLEFT ; if so, go to the screen to left on the map
LET X = RIGHTEDGE ; and put me at the right edge of that screen
EXIT ; and stop processing this event
ELSE ; if I'm not at the left edge
IF CANGOLEFT ; is there space to the left of me?
SPRITELEFT ; if so move me left
ANIMATE ; and animate me
ELSE
DIG LEFT
ENDIF
ENDIF
ENDIF
IF KEY RIGHT ; Is the right key pressed?
LET DIRECTION = RIGHT ; if so, remember I am going RIGHT
LET IMAGE = 0 ; display the right facing image
IF X >= RIGHTEDGE ; Am I at the right edge of the screen?
SCREENRIGHT ; if so go to the screen to right on the map
LET X = LEFTEDGE ; and put me at the left edge
EXIT ; and stop processing this event
ELSE ; if I'm not at the right edge
IF CANGORIGHT ; is there space to the right of me?
SPRITERIGHT ; if so, move me right
ANIMATE ; and animate me?
ELSE
DIG RIGHT
ENDIF
ENDIF
ENDIF
IF KEY UP ; is the UP key pressed?
ANIMATE SLOW ; if so, animate me slowly
IF LADDERABOVE ; is there a ladder above me?
IF CANGOUP ; can I go up?
LET IMAGE = 3 ; display the image of me climbing
SPRITEUP ; move me up
LET DIRECTION = UP ; remember I am going up
ELSE ; if there is a ladder above me, but I cant go up
ADD 1 TO Y ; nudge me 1 pixel to the right
ENDIF
ELSE ; if there isn't a ladder above me
SPRITELEFT ; move me left
IF LADDERABOVE ; now is there a ladder above me?
ELSE ; if not
SPRITERIGHT ; move me back where I was
SPRITERIGHT ; then a bit more to the right
IF LADDERABOVE ; now is there a ladder above me?
ELSE ; if not
SPRITELEFT ; move me back where I was
ENDIF
ENDIF
ENDIF
ENDIF
IF KEY DOWN ; is the DOWN key pressed?
ANIMATE SLOW ; if so, animate me slowly
IF LADDERBELOW ; is there a ladder below me?
LET IMAGE = 3 ; if so, set my image to the climbing one
SPRITEDOWN ; move me down
LET DIRECTION = DOWN ; remember I am going down
ELSE ; if there isn't a ladder below me
SPRITELEFT ; move me left
IF LADDERBELOW ; is there a ladder below me now?
ELSE ; if not
SPRITERIGHT ; move me back where I was
SPRITERIGHT ; then a bit more to the right
IF LADDERBELOW ; now is there a ladder below me?
ELSE ; if not
SPRITELEFT ; move me back where I was
ENDIF
ENDIF
ENDIF
ENDIF
IF KEY FIRE ; is the Fire key being pressed?
JUMP 7 ; if so, make me Jump to height 7
ENDIF
ENDIF ; end of the movement code
IF KEY FIRE2 ; is the FIRE2 key being pressed?
IF F = 0 ; if there is no fart in play
IF DIRECTION = LEFT ; if Stinky Dog is facing Left
ADD 16 TO X ; set X 16 pixels to the right
SPAWN 2 4 ; SPAWN a fart (TYPE 2, IMAGE 4)
SUBTRACT 16 FROM X ; reset X to its original position
LET F = 1 ; there is now a fart in play
ELSE
IF DIRECTION = RIGHT ; if Stinky Dog is facing Right
SUBTRACT 16 FROM X ; set X 16 pixels to the left
SPAWN 2 4 ; SPAWN a fart (TYPE 2, IMAGE 4)
ADD 16 TO X ; reset X to its original position
LET F = 1 ; there is now a fart in play
ENDIF
ENDIF
ENDIF
ENDIF
FALL ; make me fall if there is nothing below me
IF DEADLY ; am I touching something deadly?
KILL ; if so, kill me
ENDIF
GETBLOCKS ; if I touch any collectible blocks, run the Collected Block script
WATERBLOCKS
As of MPAGD 0.7.10 WATERBLOCKs have not been implemented fully, you can create waterblocks but they wont have any special features, they'll be like EMPTYBLOCKS. When they get implemented fully I will update this tutorial. NEXT: PART 20: SCOREs, LIVES and DISPLAYing stuff.
Comments